Query data
This page describes how to query data from QuestDB using different programming languages and tools.
For ad-hoc SQL queries, including CSV download and charting use the web console. Applications can choose between the HTTP REST API which returns JSON or use the PostgreSQL wire protocol.
Here are all your options:
- Web Console
- SQL
SELECT
statements. - Download query results as CSV.
- Chart query results.
- SQL
- PostgreSQL wire protocol
- SQL
SELECT
statements. - Use
psql
on the command line. - Interoperability with third-party tools and libraries.
- SQL
- HTTP REST API
- SQL
SELECT
statements as JSON or CSV. - Result paging.
- SQL
#
Web ConsoleQuestDB ships with an embedded Web Console running by default on port 9000
.
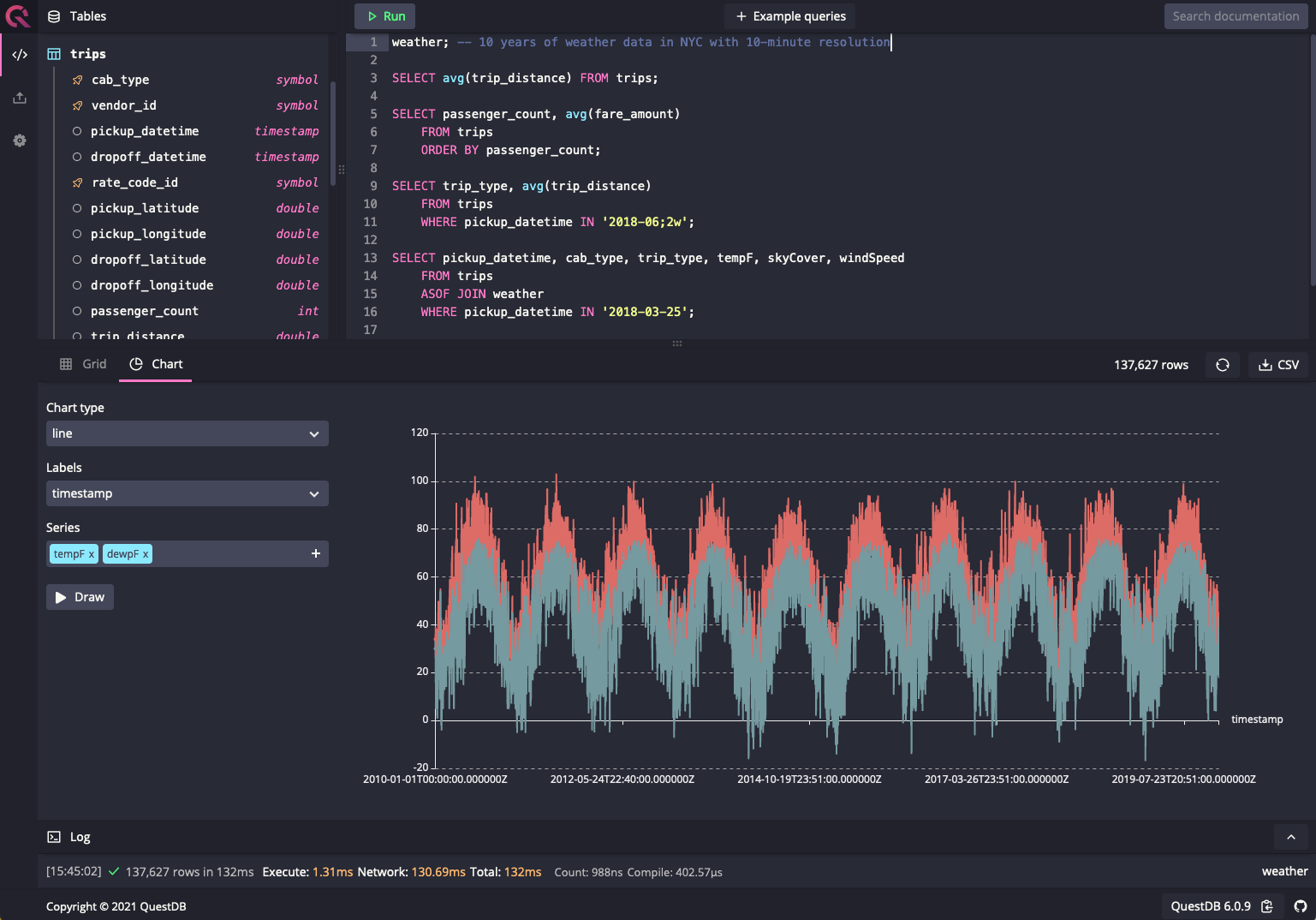
To query data from the web console, SQL statements can be written in the code editor and executed by clicking the Run button.
Aside from the Code Editor, the Web Console includes a data visualization panel for viewing query results as tables or graphs and an Import tab for uploading datasets as CSV files. For more details on these components and general use of the console, see the Web Console page.
#
PostgreSQL wire protocolYou can query data using the Postgres endpoint
that QuestDB exposes which is accessible by default via port 8812
. Examples in
multiple languages are shown below. To learn more, check out our docs about
Postgres compatibility and tools.
- Python
- Java
- NodeJS
- Go
- C#
- C
- Ruby
- PHP
#
HTTP REST APIQuestDB exposes a REST API for compatibility with a wide range of libraries and
tools. The REST API is accessible on port 9000
and has the following
query-capable entrypoints:
Entrypoint | HTTP Method | Description | API Docs |
---|---|---|---|
/exp?query=.. | GET | Export SQL Query as CSV | Reference |
/exec?query=.. | GET | Run SQL Query returning JSON result set | Reference |
For details such as content type, query parameters and more, refer to the REST API docs.
/exp
: SQL Query to CSV#
The /exp
entrypoint allows querying the database with a SQL select query and
obtaining the results as CSV.
For obtaining results in JSON, use /exec
instead, documented next.
- cURL
- Python
/exec
: SQL Query to JSON#
The /exec
entrypoint takes a SQL query and returns results as JSON.
This is similar to the /exec
entry point which returns results as CSV.
#
Querying Data- cURL
- Python
- NodeJS
- Go
The JSON response contains the original query, a "columns"
key with the schema
of the results, a "count"
number of rows and a "dataset"
with the results.